HotSpot Memory Structure
Weak generational hypothesis
When Eden Space is filled up. During GC referenced objects are moved to survivor space and un referenced are kept in memory.
Object Aging : At the next minor GC, the same thing happens for the eden space. Unreferenced objects are deleted and referenced objects are moved to a survivor space. However, in this case, they are moved to the second survivor space (S1). In addition, objects from the last minor GC on the first survivor space (S0) have their age incremented and get moved to S1. Once all surviving objects have been moved to S1, both S0 and eden are cleared. Notice we now have differently aged object in the survivor space. (thats why two different Survivor Spaces)
Promotion : when the GC count for certain objects increase from threshold ( here 8 ) its promoted
Concurrent Mark and Sweep

Types of GC
Left Side : Concurrent : Application code is running parallel to GC.
Right Side : Serial

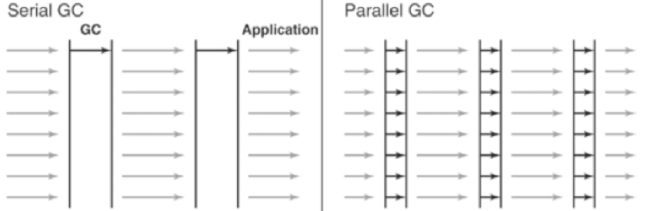
What Causes Objects to Move from Young Generation to Old Generation ?
Ans : Either of Young or Old GC , is responsible for object to move from young gen to old gen
https://stackoverflow.com/questions/39932939/what-cause-objects-to-move-from-young-generation-to-old-generation
Summary:
Weak generational hypothesis
- most objects die young
- reference from old objects to young is very small.( example : map )
When Eden Space is filled up. During GC referenced objects are moved to survivor space and un referenced are kept in memory.
Object Aging : At the next minor GC, the same thing happens for the eden space. Unreferenced objects are deleted and referenced objects are moved to a survivor space. However, in this case, they are moved to the second survivor space (S1). In addition, objects from the last minor GC on the first survivor space (S0) have their age incremented and get moved to S1. Once all surviving objects have been moved to S1, both S0 and eden are cleared. Notice we now have differently aged object in the survivor space. (thats why two different Survivor Spaces)
Promotion : when the GC count for certain objects increase from threshold ( here 8 ) its promoted
Concurrent Mark and Sweep
- Initial Mark: The application threads are paused in order to collect their object references. When this is finished, the application threads are started again.
- Concurrent Mark: Starting from the object references collected in phase 1, all other referenced objects are traversed.
- Concurrent Preclean: Changes to object references made by the application threads while phase 2 was running are used to update the results from phase 2.
- Remark: As phase 3 is concurrent as well, further changes to object references may have happened. Therefore, the application threads are stopped once more to take any such updates into account and ensure a correct view of referenced objects before the actual cleaning takes place. This step is essential because it must be avoided to collect any objects that are still referenced.
- Concurrent Sweep: All objects that are not referenced anymore get removed from the heap.
Concurrent Reset: The collector does some housekeeping work so that there is a clean state when the next GC cycle starts.
Types of GC
Left Side : Concurrent : Application code is running parallel to GC.
Right Side : Serial
Ques : Are young GC always stop world ?
For OpenJDK, JRockit, IBM JVM, and Sun/Oracle JDK, the young collection is always stop the world for every available collector. (Sep 2013) only one paid JVM has non stop world GC.
Ques : What happens when the To Space is filled ?
If the To space becomes full, the live objects from Eden or From that have not been copied to it are tenured, regardless of how many young generation collections they have survived. Any objects remaining in Eden or the From space after live objects have been copied are, by definition, not live, and they do not need to be examined.
Young generation sizing is important...
- If the young generation is too small, short-lived objects will quickly be moved into the old generation where they are harder to collect. Conversely,
- if the young generation is too large, we will have lots of unnecessary copying for long-lived objects that will later be moved to the old generation anyway.
What Causes Objects to Move from Young Generation to Old Generation ?
Ans : Either of Young or Old GC , is responsible for object to move from young gen to old gen
https://stackoverflow.com/questions/39932939/what-cause-objects-to-move-from-young-generation-to-old-generation
Summary:
- First, any new objects are allocated to the eden space. Both survivor spaces start out empty.
- When the eden space fills up, a minor garbage collection is triggered
- Referenced objects are moved to the first survivor space. Unreferenced objects are deleted when the eden space is cleared.
- At the next minor GC, the same thing happens for the eden space. Unreferenced objects are deleted and referenced objects are moved to a survivor space. However, in this case, they are moved to the second survivor space (S1)
- At the next minor GC, the same process repeats. However this time the survivor spaces switch. Referenced objects are moved to S0. Surviving objects are aged. Eden and S1 are cleared.
- After a minor GC, when aged objects reach a certain age threshold (8 in this example) they are promoted from young generation to old generation.
- As minor GCs continue to occur, objects will continue to be promoted to the old generation space.
- Eventually, a major GC will be performed on the old generation which cleans up and compacts that space.
No comments:
Post a Comment